How to add code to documentation¶
Note
This page was written in the style of a How-to Guide according to The Diátaxis Framework.
This guide shows how to include code in your documentation.
Pre-requisites¶
This guide assumes your project already has a working Sphinx documentation project in a
folder called docs
and the recommended folder structure. If you don’t
have it, you can set it up with sphinx-quickstart following the tutorial Create a New Documentation Project.
There are two ways to have code in your docs:
Inline code.
Code in a separate block.
Inline code¶
If you want to include a snippet of code inside your text, you only need to surround your code with pairs of backquotes, that is, ``your_code``
. So if you want to say that the output of 2>3
is False
, what you write is:
the output of ``2>3`` is ``False``
Code blocks¶
There are several ways to include code cells in your documentation:
Executable cells with
jupyter-execute
.Non-executable cells with
code-block
.Testable code cells with
sphinx.ext.doctest
.
Executable cells¶
If you want to run Python code that includes visualization, you can use jupyter-execute
to include cells that are executed in a Jupyter kernel and show the output of that code in your documentation. The syntax is:
.. jupyter-execute::
your_code
For example, you can write this:
.. jupyter-execute::
from qiskit import QuantumCircuit
qc = QuantumCircuit(2)
qc.h(0)
qc.cx(0,1)
qc.draw('mpl')
The output would be this cell:
from qiskit import QuantumCircuit
qc = QuantumCircuit(2)
qc.h(0)
qc.cx(0,1)
qc.draw('mpl', style='iqp')
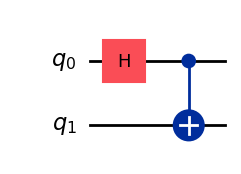
Note
Even though jupyter-execute
can be used for cells without any visualization, it makes the documentation building process
more complex, so it is recommended to use sphinx.ext.doctest
instead. See this page for more details.
Note
For the jupyter-execute
cells to appear you need to add jupyter_sphinx
to the extensions
variable in the docs/conf.py
of your repository.
Non-executable cells¶
There are some situations in which executing the code is not convenient, such as when:
The code you are showing is written in languages other than Python, like reStructuredText, YAML, Markdown or Rust.
The code requires connecting to a provider.
The code takes too long to run.
In those cases, you can use code-block
, whose syntax is:
.. code-block:: language
your_code
You can pick as language
any of the short names of the lexers supported by Pygments, like python
, bash
or text
.
For example, you can write this:
.. code-block:: python
from qiskit import QuantumCircuit
qc = QuantumCircuit(1)
qc.x(0)
And the output will look like this:
from qiskit import QuantumCircuit
qc = QuantumCircuit(1)
qc.x(0)
Testable cells¶
If you want to write Python code cells that don’t include visualizations and check if they work as intended, you have two different options:
Note
For the doctest
, testcode
and testoutput
cells to appear you need to add the extension sphinx.ext.doctest
to the conf.py
of your repository.
doctest
¶
If you want both input and output in the same code cell, you can use doctest
, whose syntax is:
.. doctest::
>>> your_code
expected_output
That way, doctest
runs your_code
and checks whether the output is expected_output
.
As an example, you can write this:
.. doctest::
>>> print(3+2)
5
Then this cell would be run:
>>> print(3+2)
5
testcode
and testoutput
¶
If you prefer to keep the code to test from the expected output, you can put the former in a testcode
cell and the latter in a testoutput
cell.
The syntax would then be:
.. testcode::
your_code
.. testoutput::
expected_output
For example, if you run this:
.. testcode::
print(3+2)
.. testoutput::
5
The output is then:
print(3+2)
5
Run the tests¶
In order to run the tests, you can use sphinx-build by setting the builder (-b
)
to doctest
:
sphinx-build -b doctest your_files output_file_path
For example, to run the tests from the docs_guide
folder and put the output.txt
file in docs_guide/_build
you can run:
sphinx-build -b doctest docs_guide docs_guide/_build
And the output will be:
Document: how_to/add_code
-------------------------
1 items passed all tests:
2 tests in default
2 tests in 1 items.
2 passed and 0 failed.
Test passed.
Doctest summary
===============
2 tests
0 failures in tests
0 failures in setup code
0 failures in cleanup code
build succeeded.
Testing of doctests in the sources finished, look at the results in docs_guide/_build/output.txt.
Add setup cells¶
For both doctest
and testcode
- testoutput
you can also add a cell that is executed before the test but not shown. This testsetup
cell can be useful,
for example, to import a package or define a function that will be used for one or more tests.
The general syntax is:
.. testsetup::
setup_code
.. testcode::
your_code
.. testoutput::
expected_output
For example, you can run this:
.. testsetup::
def hello():
print("Hello")
.. doctest::
>>> hello()
"Hello"
And the result is:
>>> hello()
Hello